As we know, CSS is a stylesheet language that was introduced to give users control over the visual (presentational) aspects of websites. Although it is still under development and keeps getting new features with every iteration, it can get a bit cumbersome when you are working on big websites, especially because many properties of CSS3 require to be defined separately for each browser with its respective prefix at this time. Fortunately for us, there's something that extends on CSS and makes it much more than a stylesheet language.
LESS is a CSS preprocessor made by Alexis Sellier, also known as cloudhead. It extends on CSS and adds features that are only found in programming languages. With LESS, you can make use of variables, functions, mixins, nesting rules, operations, color functions, etc. and reuse properties of classes and id's. And the most amazing thing about LESS is that it is backwards compatible, i.e., in addition to using LESS, you can fallback to normal CSS whenever you feel like it.
Using LESS is simple, you just need to create your .less file and link it to your website using rel="stylesheet/less" as attribute.
Client Side Usage
Link your .less stylesheets with the rel set to “stylesheet/less”:
Then download less.js from the top of the page, and include it in the <head> element of your page, like so:
Make sure you include your stylesheets before the script.
The Language
Variables
These are pretty self-explanatory:
Output:
Mixins
In LESS, it is possible to include a bunch of properties from one ruleset into another ruleset. So say we have the following class:
And we want to use these properties inside other rulesets. Well, we just have to drop in the name of the class in any ruleset we want to include its properties, like so:
The properties of the .bordered class will now appear in both #menu a and .post a:
Parametric Mixins
LESS has a special type of ruleset which can be mixed in like classes, but accepts parameters. Here’s the canonical example:
And here’s how we can mix it into various rulesets:
Parametric mixins can also have default values for their parameters:
We can invoke it like this now:
And it will include a 5px border-radius.
You can also use parametric mixins which don’t take parameters. This is useful if you want to hide the ruleset from the CSS output, but want to include its properties in other rulesets:
Which would output:
The @arguments variable
@arguments has a special meaning inside mixins, it contains all the arguments passed, when the mixin was called. This is useful if you don’t want to deal with individual parameters:
Which results in:
Any CSS class or id ruleset can be mixed-in that way.
Nested rules
LESS gives you the ability to use nesting instead of, or in combination with cascading. Lets say we have the following CSS:
In LESS, we can also write it this way:
Or this way:
The resulting code is more concise, and mimics the structure of your DOM tree.
Notice the & combinator—it’s used when you want a nested selector to be concatinated to its parent selector, instead of acting as a descendent. This is especially important for pseudo-classes like :hover and :focus.
For example:
Will output
Operations
Any number, color or variable can be operated on. Here are a couple of examples:
The output is pretty much what you expect—LESS understands the difference between colors and units. If a unit is used in an operation, like in:
LESS will use that unit for the final output—6px in this case.
Brackets are also authorized in operations:
And are required in compound values:
Color functions
LESS provides a variety of functions which transform colors. Colors are first converted to the HSL color-space, and then manipulated at the channel level:
Using them is pretty straightforward:
You can also extract color information:
This is useful if you want to create a new color based on another color’s channel, for example:
@new will have @old’s hue, and its own saturation and lightness.
Math functions
LESS provides a couple of handy math functions you can use on number values:
If you need to turn a value into a percentage, you can do so with the percentage function:
For a complete list of features check the official website (http://lesscss.org)
Still not convinced enough to make the transition from classic CSS?
If you like the features of LESS but don't want to use LESS directly then there's a solution for that. SimpLESS is a cross-platform LESS to CSS compiler. You don't have to do any extra work to compile your LESS files to CSS. Just add your LESS stylesheets or project folders to SimpLESS by dragging and dropping and it would automatically create an updated CSS stylesheet every time you makes changes to your LESS file.
Get SimpLESS from here:
http://wearekiss.com/simpless
Other options - online LESS compilers:
http://http://lesstester.com
http://winless.org/online-less-compiler
Most of the information on this thread has been sourced from LESS' official website. For more info visit [Official Website] : http://lesscss.org
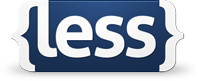
LESS is a CSS preprocessor made by Alexis Sellier, also known as cloudhead. It extends on CSS and adds features that are only found in programming languages. With LESS, you can make use of variables, functions, mixins, nesting rules, operations, color functions, etc. and reuse properties of classes and id's. And the most amazing thing about LESS is that it is backwards compatible, i.e., in addition to using LESS, you can fallback to normal CSS whenever you feel like it.
Using LESS is simple, you just need to create your .less file and link it to your website using rel="stylesheet/less" as attribute.
Client Side Usage
Link your .less stylesheets with the rel set to “stylesheet/less”:
Code:
<link rel="stylesheet/less" type="text/css" href="styles.less">
Code:
<script src="less.js" type="text/javascript"></script>
The Language
Variables
These are pretty self-explanatory:
Code:
@nice-blue: #5B83AD;
@light-blue: @nice-blue + #111;
#header { color: @light-blue; }
Code:
#header { color: #6c94be; }
In LESS, it is possible to include a bunch of properties from one ruleset into another ruleset. So say we have the following class:
Code:
.bordered {
border-top: dotted 1px black;
border-bottom: solid 2px black;
}
Code:
#menu a {
color: #111;
.bordered;
}
.post a {
color: red;
.bordered;
}
Code:
#menu a {
color: #111;
border-top: dotted 1px black;
border-bottom: solid 2px black;
}
.post a {
color: red;
border-top: dotted 1px black;
border-bottom: solid 2px black;
}
LESS has a special type of ruleset which can be mixed in like classes, but accepts parameters. Here’s the canonical example:
Code:
.border-radius (@radius) {
border-radius: @radius;
-moz-border-radius: @radius;
-webkit-border-radius: @radius;
}
Code:
#header {
.border-radius(4px);
}
.button {
.border-radius(6px);
}
Code:
.border-radius (@radius: 5px) {
border-radius: @radius;
-moz-border-radius: @radius;
-webkit-border-radius: @radius;
}
Code:
#header {
.border-radius;
}
You can also use parametric mixins which don’t take parameters. This is useful if you want to hide the ruleset from the CSS output, but want to include its properties in other rulesets:
Code:
.wrap () {
text-wrap: wrap;
white-space: pre-wrap;
white-space: -moz-pre-wrap;
word-wrap: break-word;
}
pre { .wrap }
Code:
pre {
text-wrap: wrap;
white-space: pre-wrap;
white-space: -moz-pre-wrap;
word-wrap: break-word;
}
@arguments has a special meaning inside mixins, it contains all the arguments passed, when the mixin was called. This is useful if you don’t want to deal with individual parameters:
Code:
.box-shadow (@x: 0, @y: 0, @blur: 1px, @color: #000) {
box-shadow: @arguments;
-moz-box-shadow: @arguments;
-webkit-box-shadow: @arguments;
}
.box-shadow(2px, 5px);
Code:
box-shadow: 2px 5px 1px #000;
-moz-box-shadow: 2px 5px 1px #000;
-webkit-box-shadow: 2px 5px 1px #000;
Nested rules
LESS gives you the ability to use nesting instead of, or in combination with cascading. Lets say we have the following CSS:
Code:
#header { color: black; }
#header .navigation {
font-size: 12px;
}
#header .logo {
width: 300px;
}
#header .logo:hover {
text-decoration: none;
}
Code:
#header {
color: black;
.navigation {
font-size: 12px;
}
.logo {
width: 300px;
&:hover { text-decoration: none }
}
}
Code:
#header { color: black;
.navigation { font-size: 12px }
.logo { width: 300px;
&:hover { text-decoration: none }
}
}
Notice the & combinator—it’s used when you want a nested selector to be concatinated to its parent selector, instead of acting as a descendent. This is especially important for pseudo-classes like :hover and :focus.
For example:
Code:
.bordered {
&.float {
float: left;
}
.top {
margin: 5px;
}
}
Code:
.bordered.float {
float: left;
}
.bordered .top {
margin: 5px;
}
Any number, color or variable can be operated on. Here are a couple of examples:
Code:
@base: 5%;
@filler: @base * 2;
@other: @base + @filler;
color: #888 / 4;
background-color: @base-color + #111;
height: 100% / 2 + @filler;
Code:
@var: 1px + 5;
Brackets are also authorized in operations:
Code:
width: (@var + 5) * 2;
Code:
border: (@width * 2) solid black;
LESS provides a variety of functions which transform colors. Colors are first converted to the HSL color-space, and then manipulated at the channel level:
Code:
lighten(@color, 10%); // return a color which is 10% *lighter* than @color
darken(@color, 10%); // return a color which is 10% *darker* than @color
saturate(@color, 10%); // return a color 10% *more* saturated than @color
desaturate(@color, 10%); // return a color 10% *less* saturated than @color
fadein(@color, 10%); // return a color 10% *less* transparent than @color
fadeout(@color, 10%); // return a color 10% *more* transparent than @color
fade(@color, 50%); // return @color with 50% transparency
spin(@color, 10); // return a color with a 10 degree larger in hue than @color
spin(@color, -10); // return a color with a 10 degree smaller hue than @color
mix(@color1, @color2); // return a mix of @color1 and @color2
Code:
@base: #f04615;
.class {
color: saturate(@base, 5%);
background-color: lighten(spin(@base, 8), 25%);
}
Code:
hue(@color); // returns the `hue` channel of @color
saturation(@color); // returns the `saturation` channel of @color
lightness(@color); // returns the 'lightness' channel of @color
alpha(@color); // returns the 'alpha' channel of @color
Code:
@new: hsl(hue(@old), 45%, 90%);
Math functions
LESS provides a couple of handy math functions you can use on number values:
Code:
round(1.67); // returns `2`
ceil(2.4); // returns `3`
floor(2.6); // returns `2`
Code:
percentage(0.5); // returns `50%`
Still not convinced enough to make the transition from classic CSS?
If you like the features of LESS but don't want to use LESS directly then there's a solution for that. SimpLESS is a cross-platform LESS to CSS compiler. You don't have to do any extra work to compile your LESS files to CSS. Just add your LESS stylesheets or project folders to SimpLESS by dragging and dropping and it would automatically create an updated CSS stylesheet every time you makes changes to your LESS file.
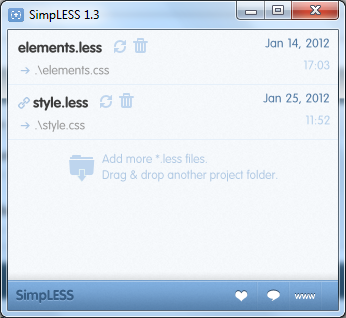
Get SimpLESS from here:
http://wearekiss.com/simpless
Other options - online LESS compilers:
http://http://lesstester.com
http://winless.org/online-less-compiler
Most of the information on this thread has been sourced from LESS' official website. For more info visit [Official Website] : http://lesscss.org
Last edited: